Getting Email API Key/Credentials
Resend
Go to Resend and log in or create and account if you haven't. Once you log in, you will be redirected to the email page.
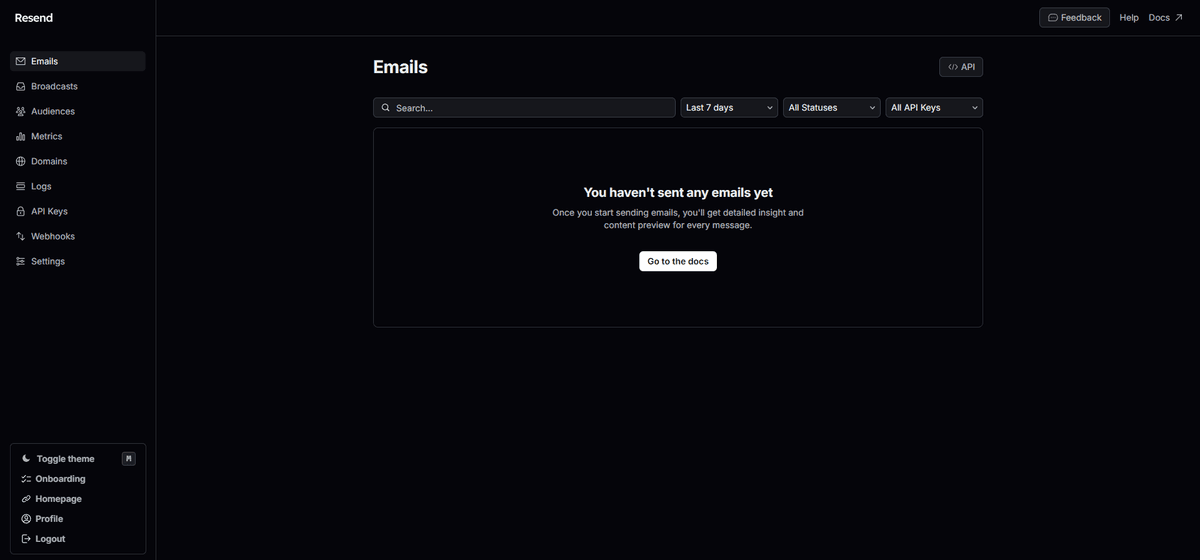
Click on the three dots on the bottom left where your email is showing, and go to onboarding.
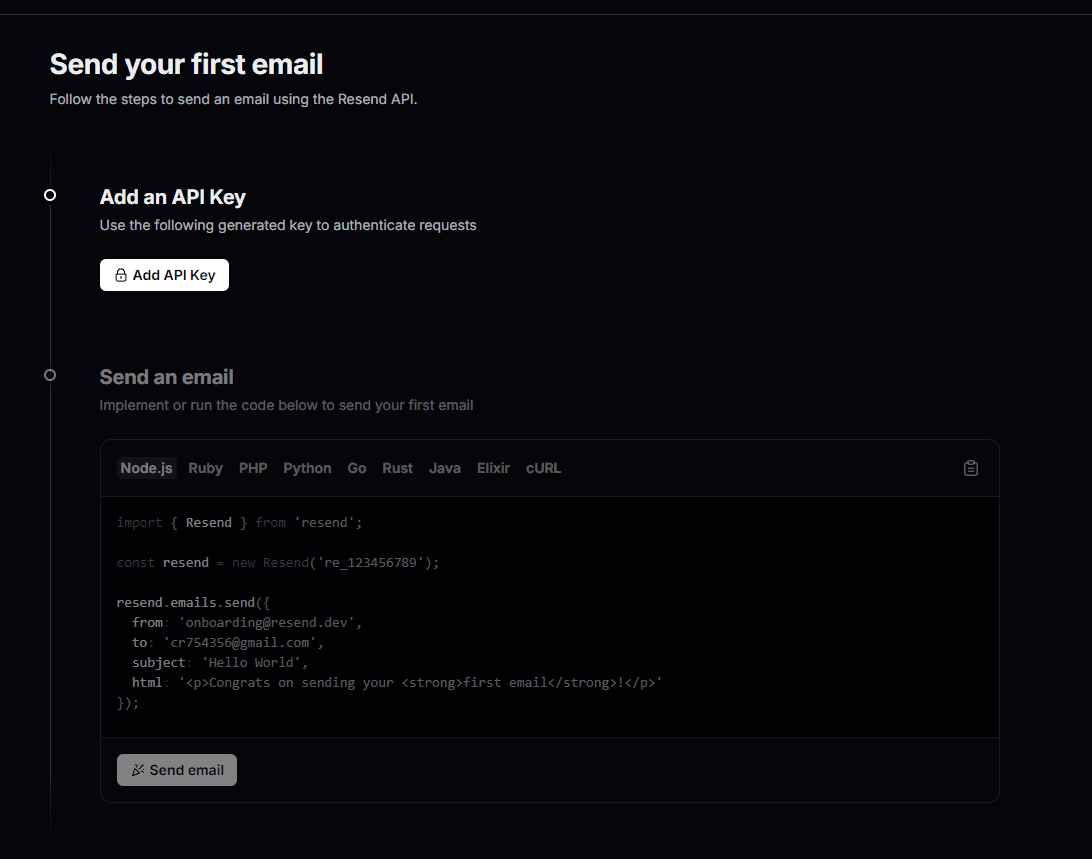
Click on Add an API Key. Copy the key and paste it in your .env file.
After adding the api key, open your local server and install resend.
Go to mail.ts file. Delete the nodemailer related stuff and add the following code.
To send emails you have to change only one thing. I'll explain by changing sendVerificationEmail from nodemailer to resend.
Nodemailer
Note: I have change the backticks to '' due to an issue here.
Resend
Note: I have change the backticks to '' due to an issue here.
You just have to change the starting code e.g., await resend.emails.send and the from email. You can add your email once you add a .com domain in resend dashboard. Also the email will only be sent to you. To make it work for others as well, you have to add a .com domain.